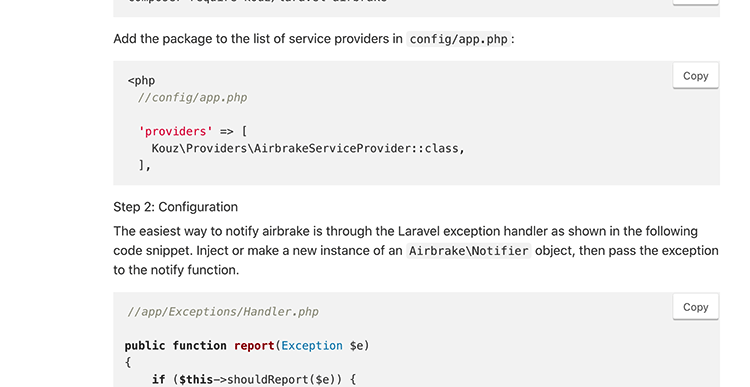
It's critical for us to be notified of any errors that occur on our production websites.
It's also extremely useful to be able to search through all our server logs, either to find the cause of an error, or just to keep an eye on things.
After using a large number of systems over the years, this is the configuration we use for all our bigger Laravel projects. We've settled on two third-party services: Airbrake, for error monitoring, and Papertrail, for log file analysis.
Airbrake
There's a wide variety of services that are designed to capture and store errors from a web application; Sentry, Rollbar, BugSnag, Raygun and many more.
However, having tried all of the above, our favourite is Airbrake.
Setting up an account is straightforward; simply visit https://airbrake.io/ and click "Get Started". They request a few personal details, and then invite you to create your first project.
Note that when you first create a project in Airbrake and indicate that you're using Laravel, you're directed to this page:
The instructions on this page are incorrect, and should be disregarded!
If you search for documentation on the main Airbrake website, you might find this page instead:
https://airbrake.io/docs/installing-airbrake/installing-airbrake-in-a-laravel-application/
This page is also incorrect! Ignore it.
This doesn't bode well, but in fact, Airbrake is excellent, and works very well once it's installed correctly. The correct instructions (as of 10/06/2020) are here:
https://github.com/TheoKouzelis/laravel-airbrake
We won't duplicate the instructions here, but they're very simple to follow. Note that you will be adding 'airbrake' to your log 'stack'; see below for more information on this.
Also, Airbrake (as opposed to other similar services, such as Rollbar) doesn't seem to log events that are created using Laravel's Log::
facade. However, we don't find that this is an issue; we prefer to use Airbrake for actual errors, and Papertrail to review log messages.
Papertrail
Papertrail essentially hosts your log files in the cloud. This is handy if you're running a container-based server (where local storage is overwritten when the server is rebuilt), but it's also much easier on a standard server. Rather than interrogate static log files using grep or similar, you can quickly and easily search through your logs using the Papertrail web interface.
Papertrail is extremely easy to integrate with Laravel. Create an account at papertrailapp.com, and you'll be given a URL and project number. For example, logs5.papertrailapp.com
and 12345
. You then add them to the "papertrail" array in config/logging.php
:
'papertrail' => [
'driver' => 'monolog',
'level' => 'debug',
'handler' => SyslogUdpHandler::class,
'handler_with' => [
'host' => 'logs5.papertrailapp.com',
'port' => '12345'
],
],
}
You can then add "papertrail" to your log stack (see below).
Logfiles
Having static log files may still be useful. Whether you use a 'daily' file (where Laravel creates individual log files each day), or a 'single' file (ie one huge log file that grows over time) is up to you. Either way, you add the relevant interval to your log stack (see below).
The log stack
This is where you tell your application how to log all errors. If you wish to use Airbrake, and Papertrail, and individual daily log files, your config/logging.php file will look like this:
{
'channels' => [
'stack' => [
'driver' => 'stack',
'channels' => ['airbrake', 'papertrail', 'daily'],
'ignore_exceptions' => false,
],
}
Done
That's it! Once this code is in place, Airbrake will notify you of any new errors that occur, and Papertrail will store all your log files in the cloud. Your logs will remain on your server, just in case.
A bonus tip
Laravel can struggle with log permissions sometimes; the webserver might create a file that an artisan
command can't then update, or vice versa.
This can be worked around by making sure that the user account for the webserver (such as www-data
) is in the same group as your command line user, and so on. However, this can be a bit fiddly.
Instead, there's a configuration setting in Laravel that isn't documented much, but which is very helpful. When configuring your 'daily' log file (or, if you prefer to use 'single'), you can set an option for permission
, like so:
'daily' => [
'driver' => 'daily',
'path' => storage_path('logs/laravel.log'),
'level' => 'debug',
'days' => 14,
'permission' => 0666
],
Setting this option to 0666
means that the log files are writeable by any user.
Technically there could be some security issues here... but really, they're pretty minimal. It might not be wise if you share your server with other users, but really, who does that nowadays? For convenience, 0666
works very well.