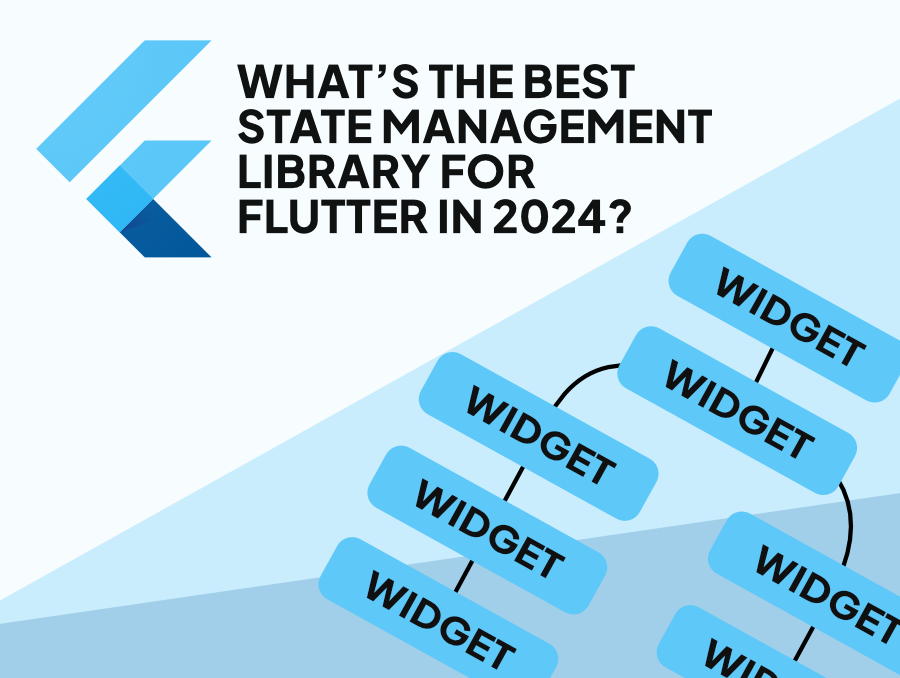
Remember that whirlwind year we just had in the Flutterverse? New trends emerged, libraries evolved, and navigating the state management landscape felt like riding a rollercoaster (a fun one, but a rollercoaster nonetheless!).
Well, fasten your seatbelts, because it's 2024, and the ride continues! In this follow-up to our 2023 article, we're diving deep once again to help you choose the perfect state management library for your next Flutter project.
Stay tuned as we explore the latest updates, compare the benefits, and unveil the best options for effective state management in the exciting year ahead!
Clean Architecture for a Smooth Ride: Why Separation Matters
Before we jump into the library showdown, let's take a step back and talk about a fundamental concept: separation of concerns. In Flutter, this means keeping your app's business logic (the "what" and "how" behind your features) separate from the presentation layer (the UI, or the "what the user sees").
Why is this so important? Imagine a tangled mess of code where UI elements and complex calculations are interwoven. Not a pretty picture, right? Separating these concerns brings a host of benefits:
Readability: Your code becomes much easier for other developers to read, making it easier for you and your team to understand and maintain.
Testability: Isolating logic lets you write unit tests with ease, ensuring your app functions flawlessly.
Flexibility: Changes to the UI won't break the logic, and vice versa. This makes your app more adaptable to future needs.
Here's where state management libraries come in as your secret weapon. They provide a structured way to manage application state, keeping it separate from your widgets.
Stateless widgets are simpler, lighter-weight, and easier to test. They simply render UI based on the data they receive, without the burden of managing state themselves. By leveraging state management libraries, you can keep your UI clean and your codebase maintainable.
Let’s take a tour through four of the most popular state management libraries, see how they work, and hopefully provide you with enough information to make an informed decision for your own Flutter app.
Provider: A Simple State Management Solution for Flutter
Provider is a popular state management solution for Flutter applications. It simplifies and streamlines the process of sharing data across different parts of your app. Here's a quick introduction and how it works:
Introduction to Provider
Lightweight: Easy to learn and implement, requiring minimal boilerplate code.
Flexible: Adapts to different needs, allowing state management for individual widgets or entire screens.
Built on InheritedWidgets: Leverages existing Flutter mechanisms for efficient data distribution.
How Provider works
ChangeNotifier: This class, included in the Flutter SDK, acts as the foundation for state management. It allows notifying any listening widgets when the state changes.
Creating State Models: You define classes extending ChangeNotifier to encapsulate your application's state. These models hold the data and methods to update it.
Provider Widgets: These widgets act as bridges, providing the state model to descendant widgets within the widget tree. Three main types are used:
ChangeNotifierProvider: Creates and manages a single instance of your state model.
MultiProvider: Combines multiple providers for managing different state models.
Consumer: A widget that listens to changes in the provided state model and rebuilds itself when the state updates.
Benefits of using Provider
Improved code maintainability: Separates state management logic from UI widgets.
Simplified data sharing: Makes data accessible throughout the widget tree easily.
Enhanced testability: Isolates state management code for easier testing.
Overall, Provider offers a good balance between simplicity and flexibility for managing state in Flutter applications, especially for small to medium-sized projects.
BLoC: Streamlined State Management for Flutter
BLoC (Business Logic Component) is a state management pattern popular in Flutter for its structured approach and separation of concerns. Here's a basic introduction and how it works:
Introduction to BLoC:
Structured flow: Utilises events, states, and a BLoC/Cubit class to manage data flow.
Separation of concerns: Keeps UI and business logic separate, improving code maintainability.
Reactive architecture: Employs streams to update the UI automatically based on state changes.
How BLoC works
Events: Represent user interactions or other actions triggering state changes. They are typically data classes capturing the necessary information for the BLoC to act upon.
BLoC Class: Acts as the central component, responsible for:
Processing logic: Performs computations and data manipulation based on the received event.
Emitting output: Generates new states through a StateStream.
States: Represent the current state of your application data. They are immutable data objects reflecting the changes triggered by events.
UI: Listens to the BLoC's StateStream and rebuilds itself when a new state is emitted, ensuring the UI always reflects the current state.
Benefits of using BLoC
Improved code organisation: Promotes a cleaner and more modular codebase.
Enhanced testability: Isolates business logic in the BLoC, making it easier to test.
Scalability: Well-suited for complex applications with multiple data sources.
Overall, BLoC offers a structured and reactive approach to state management, ideal for projects requiring clear separation of concerns and good testability.
Riverpod: State Management Evolution in Flutter
Riverpod is a relatively new and powerful state management solution for Flutter, built upon the foundation of Provider. It aims to address some of Provider's limitations and offers additional features:
Introduction to Riverpod
An Enhanced Provider: Extends and improves Provider's functionality.
Reactive state management: Offers automatic rebuilds on state changes.
Dependency injection: Provides a clean and organised way to manage dependencies.
Testing-friendly: Supports easy mocking and isolation for unit tests.
How Riverpod works
Providers: Similar to Provider, Riverpod uses providers to encapsulate data and logic. These can be:
State Providers: Store mutable state using the StateNotifierProvider and update it with methods.
Provider: Holds immutable data or instances of classes like network clients.
Hooks: Riverpod introduces hooks like useProvider and useWatch to access and interact with providers within widgets.
Dependency Injection: Providers become the single source of truth for dependencies, making them readily available throughout the widget tree.
Benefits to using Riverpod
Simpler and more concise API: Easier to learn and less verbose compared to Provider.
Better performance: Optimises data and widget rebuilds for improved efficiency.
Advanced features: Offers features like family providers for dynamic data handling.
Overall, Riverpod offers a modern and streamlined approach to state management, aiming for improved ease of use, performance, and testability. It's a good choice for both beginners and experienced developers seeking a robust and efficient solution.
MobX: Reactive Simplicity for State Management in Flutter
MobX empowers you to manage application state in Flutter with a reactive and intuitive approach. It emphasises simplicity and ease of use, allowing you to focus on your app's core functionality.
How MobX Works
Define Observables: Declare your application's state as observable variables using the @observable decorator. These variables automatically notify any listening widgets when their values change.
Create Actions: Encapsulate state modifications within actions, denoted by the @action decorator. This ensures predictable and controlled state updates.
Utilise Reactions: Leverage reactions to automatically execute code whenever an observable changes. You define them using the autorun function, specifying the code to run and the observables to listen to.
Wrap with Observer: Wrap your UI widgets with the Observer widget from the mobx_flutter package. This widget automatically rebuilds the UI whenever a relevant observable changes, ensuring your UI always reflects the current state.
Benefits of using MobX
Simple and intuitive: Requires minimal boilerplate code for quick setup.
Reactive approach: Automates UI updates based on state changes, reducing manual work.
Easy debugging: Clear separation of state and UI logic simplifies debugging.
MobX shines when you prioritise clean and concise code. Its minimal boilerplate code keeps your codebase easy to understand and maintain. This translates to a smoother development experience, allowing you to focus on building features rather than wrestling with complex state management logic. Additionally, MobX's simplicity makes it well-suited for smaller to medium-sized projects, offering a good balance between ease of use and efficiency.
GetX: Power Up Your Flutter State Management with Ease
GetX is a powerful yet lightweight state management solution for Flutter. It goes beyond just state management, offering a complete ecosystem that simplifies various aspects of development.
How GetX Works
State Management with Controllers: Create dedicated classes called controllers to manage your application state. These controllers hold your data and methods to update it.
Dependency Injection: Inject your controllers and other dependencies directly into your widgets using intuitive syntax. This eliminates the need for complex provider trees and makes your code cleaner.
Automatic UI Updates: Utilise the Obx widget to automatically rebuild your UI whenever the underlying state in your controller changes. No more manual rebuild logic needed!
Easier Navigation: Take advantage of GetX's built-in navigation features to manage navigation flows within your app with ease. It provides a simple API for defining routes and transitions.
Benefits of using GetX
Increased developer productivity: Write less code with GetX's concise syntax and built-in features.
Improved code organisation: Separate state management logic from UI widgets for better maintainability.
Enhanced performance: GetX is optimised for efficiency, ensuring smooth app responsiveness.
If you’re looking for a more comprehensive solution for state management GetX might be the perfect fit. It offers a complete ecosystem encompassing not just state management, but also dependency injection and navigation. This all-in-one approach simplifies your development process, allowing you to focus on building features rather than managing various separate libraries.
Conclusion
Remember, the best library for your project depends on its specific needs and your team's preferences. Consider factors like project complexity, desired features, and team experience to make the optimal choice and embark on a smooth and successful Flutter development journey!